|
 |
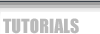 |
 |
|
Load your CLASSPATH at runtime
By Gal Ratner
One of the great advantages of programming in JAVA is the ability to add to your code as you go. You can create classes and turn them into packages which can then be imported and used in any other JAVA application. Each instance of your program relies on the CLASSPATH environment variable for external packages. For example you can import an external JDBC driver for your application to connect your database. You can also import dynamic modules to add and change program functionality. Setting the classpath variable can be done as a part in the environment variables or while invoking the JVM. However, when your program relies on external web updates, it’s not practical to change the command arguments every time there is a major update. In this article, we are going to explore programmically changing your classpath to modify program features. We are going to assume you have an updater module that basically drops all of your JAR files into the module’s directory. We are then going to read all the files in the directory and add them to your classpath at run time. The code to reading all the files in the directory looks like this:
   public static String moduleFolder = "C:\\Program Files\\Tarantula\\modules";
   File moduleDirectory = new File(moduleFolder);
   String classpath = System.getProperty("java.class.path");
   File[] moduleFiles = moduleDirectory.listFiles();
   for (int i = 0; i < moduleFiles.length; i++){
      File moduleFile = driverFiles[i];
         if (moduleFile.getName().endsWith(".jar")){
            if(classpath.indexOf(moduleFiles[i].getName()) == -1){
            try{
            addFile(moduleFiles[i]);
            }catch(IOException e){}
         }
         }else{
         moduleFile.delete();
         }
      }
   }
And the last step is to append the files to the classfile.
   public static void addFile(File moduleFile) throws IOException {
      URL moduleURL = moduleFile.toURL();
      private static final Class[] parameters = new Class[]{URL.class};
      sysloader = (URLClassLoader)ClassLoader.getSystemClassLoader();
      Class sysclass = URLClassLoader.class;
      try {
         Method method = sysclass.getDeclaredMethod("addFile",parameters);
         method.setAccessible(true);
         method.invoke(sysloader,new Object[]{ moduleURL });
      } catch (Throwable t) {
         t.printStackTrace();
         throw new IOException("Error, could not add URL to system classloader");
      }
   }
It is very important to program your classpath as the first step when you invoke your program. Any objects that are being initialized before the classpath is set can result in a no class definition error.
You’ve seen how useful it can be to any software that relies on external packages. The possibilities are endless. Happy programming!
|
|
|
 |
|
 |
About Us | Contact Us | Standards of Business Conduct | Employment
© InvertedSoftware.com All rights reserved
|